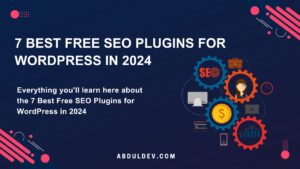
Create a Responsive Sticky Navigation Bar Using HTML, CSS, and JavaScript
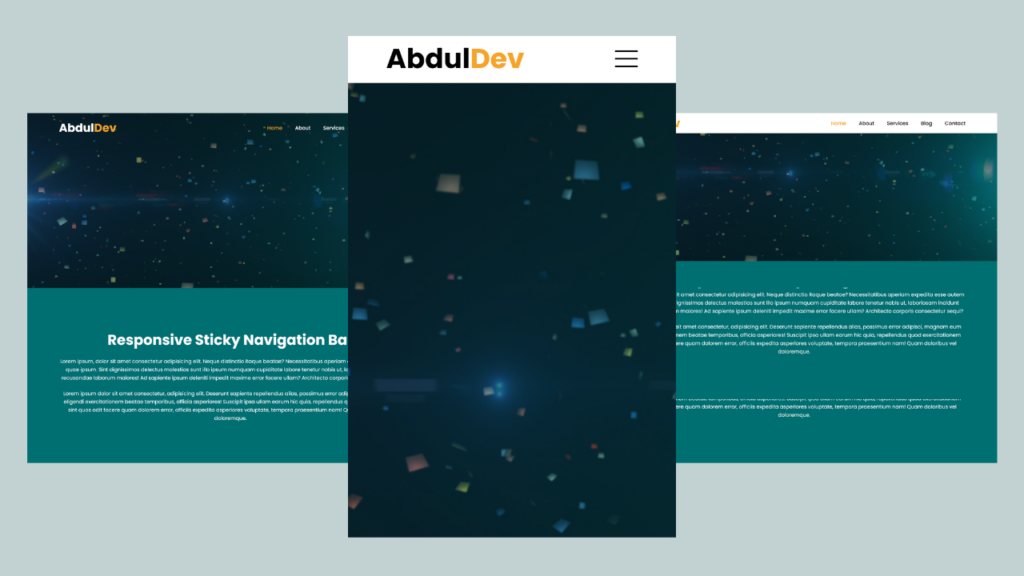
Creating a responsive sticky navigation bar is essential for modern web design. It enhances user experience by allowing easy navigation and constant access to key menu items. In this article, you’ll learn how to create a sticky navigation bar that stays at the top of the page as you scroll. We’ll provide the full source code so you can follow along and implement it in your projects.
Check Out Those Useful Articles
Prerequisites
Before we start, ensure you have a basic understanding of:
- HTML
- CSS
- JavaScript
Responsive Navigation Bar HTML, CSS and JavaScript (Free Source Code)
To create a Navigation Bar using HTML, CSS, and JavaScript, follow these steps:
- Create a folder: Name the folder anything you like. Inside this folder, create the following files:
- Create an index.html file: The file must be named index with a .html extension.
- Create a style.css file: The file must be named style with a .css extension.
- Create a main.js file: The file must be named main with a .js extension.
After creating these files, copy and paste the provided code snippets into the respective files. Alternatively, you can download the complete Navigation Bar HTML, CSS, and JavaScript files by clicking the download button below.
First, paste the following codes into your index.html file.
Responsive Sticky Navigation Bar
AbdulDev
Responsive Sticky Navigation Bar
Lorem ipsum, dolor sit amet consectetur adipisicing elit. Neque distinctio itaque beatae? Necessitatibus aperiam expedita esse autem quae ipsum. Sint dignissimos delectus molestias sunt illo ipsum numquam cupiditate labore tenetur nobis ut, laboriosam incidunt recusandae laborum maiores! Ad sapiente ipsum deleniti impedit maxime error facere ullam? Architecto corporis consectetur sequi?
Lorem ipsum dolor sit amet consectetur, adipisicing elit. Deserunt sapiente repellendus alias, possimus error adipisci, magnam eum eligendi exercitationem beatae temporibus, officia asperiores! Suscipit ipsa ullam earum hic quia, repellendus quod exercitationem sint quos odit facere quam dolorem error, officiis expedita asperiores voluptate, tempora praesentium nam! Quam doloribus vel doloremque.
Second, paste the following codes into your style.css file.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;700&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
header{
position: fixed;
top: 0;
left: 0;
width: 100%;
padding: 20px 100px;
display: flex;
justify-content: space-between;
align-items: center;
z-index: 1000;
transition: 0.5s;
}
/* Sticky CodeK */
.stickyNav{
padding: 5px 100px;
background: #fff;
}
.stickyNav .logo{
color: #000;
}
.stickyNav ul li a{
color: #000;
}
header .logo{
position: relative;
text-decoration: none;
font-size: 36px;
font-weight: 700;
transition: 0.5s;
color: #fff;
}
.logo span{
color: #f7a328;
}
header ul{
position: relative;
display: flex;
gap: 40px;
}
header ul li{
list-style: none;
}
header ul li a{
text-decoration: none;
font-size: 16px;
font-weight: 500;
transition: 0.5s;
color: #fff;
}
header ul li.active a,
header ul li:hover a{
color: #f7a328;
}
#hero{
position: relative;
width: 100%;
min-height: 100vh;
background-image: url(Hero-section1.png);
background-position: center;
background-repeat: no-repeat;
background-size: cover;
background-blend-mode: color;
background-color: #0000007a;
}
#about{
position: relative;
background: #006f72;
width: 100%;
min-height: 100vh;
padding: 120px 100px;
display: flex;
justify-content: center;
flex-direction: column;
}
#about .secText{
position: relative;
width: 100%;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
}
.secText h2{
font-size: 45px;
color: #fff;
padding-bottom: 20px;
}
.secText p{
text-align: center;
color: #fff;
font-size: 16px;
line-height: 26px;
}
/* Need to Make Responsive for all Devices */
@media screen and (max-width: 900px) {
header{
padding: 20px 50px;
}
.secText h2{
font-size: 30px;
line-height: 36px;
text-align: center;
padding-bottom: 30px;
}
#about{
padding: 100px 50px;
}
.menuToggle{
position: relative;
width: 30px;
height: 20px;
cursor: pointer;
display: flex;
justify-content: center;
align-items: center;
}
.menuToggle::before{
content: '';
position: absolute;
width: 100%;
height: 2px;
background: #fff;
transform: translateY(-10px);
box-shadow: 0 10px #fff;
transition: 0.5s;
}
.menuToggle::after{
content: '';
position: absolute;
width: 100%;
height: 2px;
background: #fff;
transform: translateY(10px);
transition: 0.5s;
}
header.active .menuToggle::before{
transform: translateY(0) rotate(45deg);
box-shadow: 0 0 #fff;
}
header.active .menuToggle::after{
transform: translateY(0) rotate(315deg);
}
header ul{
display: none;
}
header.active ul{
position: fixed;
left: 0;
top: 84px;
width: 100%;
height: calc(100vh - 84px);
background: #036960fe;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
gap: 16px;
}
header.active ul a{
font-size: 22px;
transition: 0.5s;
}
.stickyNav .menuToggle::before{
background: #000;
box-shadow: 0 10px #000;
}
.stickyNav .menuToggle::after{
background: #000;
}
.stickyNav ul.nav{
top: 60px !important;
height: calc(100vh - 60px);
}
.stickyNav ul li a{
color: #fff;
}
.stickyNav{
padding: 5px 50px;
}
}
Third, paste the following codes into your main.js file.
window.addEventListener("scroll", function (){
var header = document.querySelector("header");
header.classList.toggle("stickyNav", window.scrollY > 0);
})
let menuToggle = document.querySelector(".menuToggle");
let header = document.querySelector("header");
menuToggle.onclick = function(){
header.classList.toggle("active");
}
Conclusion
You’ve successfully created a responsive sticky navigation bar using HTML, CSS, and JavaScript. This navigation bar will enhance user experience by providing easy access to the main sections of your website at all times.
Share on Social Media
FAQs
A sticky navigation bar is a menu that remains fixed at the top of the webpage as the user scrolls down. This ensures that the navigation menu is always accessible, enhancing the user experience by allowing easy access to different sections of the website.
To make your navigation bar responsive, you can use CSS media queries to adjust the layout based on the screen size. For example, you can switch from a horizontal to a vertical layout on smaller screens. Here’s an example of how to do this:
@media screen and (max-width: 600px) {
header nav ul {
flex-direction: column;
}
header nav ul li {
margin: 10px 0;
}
}
Common reasons why your sticky navigation bar might not be working include:
- Incorrect JavaScript function or missing event listener for the scroll event.
- Missing or incorrect CSS styling for the sticky class.
- HTML elements not correctly identified in your JavaScript.
Ensure your JavaScript is correctly implemented to add and remove the sticky class based on the scroll position, and verify your HTML and CSS selectors are accurate.
You can add smooth scrolling to your navigation links using CSS or JavaScript. Here’s a simple way to do it with CSS:
html {
scroll-behavior: smooth;
}
This CSS property ensures that when users click on navigation links, the page scrolls smoothly to the corresponding section.
Yes, you can customize the appearance of your sticky navigation bar by modifying the CSS. You can change colors, add hover effects, adjust padding, and more. For example, to change the background color and add a box shadow when the navbar becomes sticky, you can use:
header.sticky {
background-color: #444;
box-shadow: 0 4px 2px -2px gray;
}
Related Articles
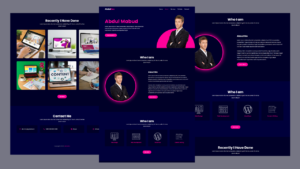
Creating a Responsive Portfolio Website with HTML, CSS, and JavaScript
Learn how to create a stunning and responsive portfolio website using HTML, CSS, and JavaScript. A step-by-step guide to showcase
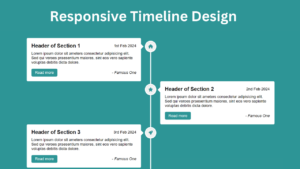
Responsive Vertical Timeline Design using HTML and CSS
Responsive Vertical Timeline Design using HTML and CSS Discover how to craft a Responsive Vertical Timeline Design utilizing HTML &
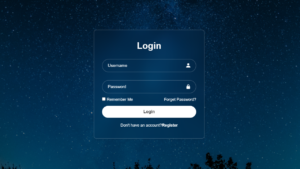
Transparent Animated Login Form Using HTML and CSS 2024
Transparent Animated Login Form Using HTML and CSS Transparent Animated Login Form is one of the best user experiences in