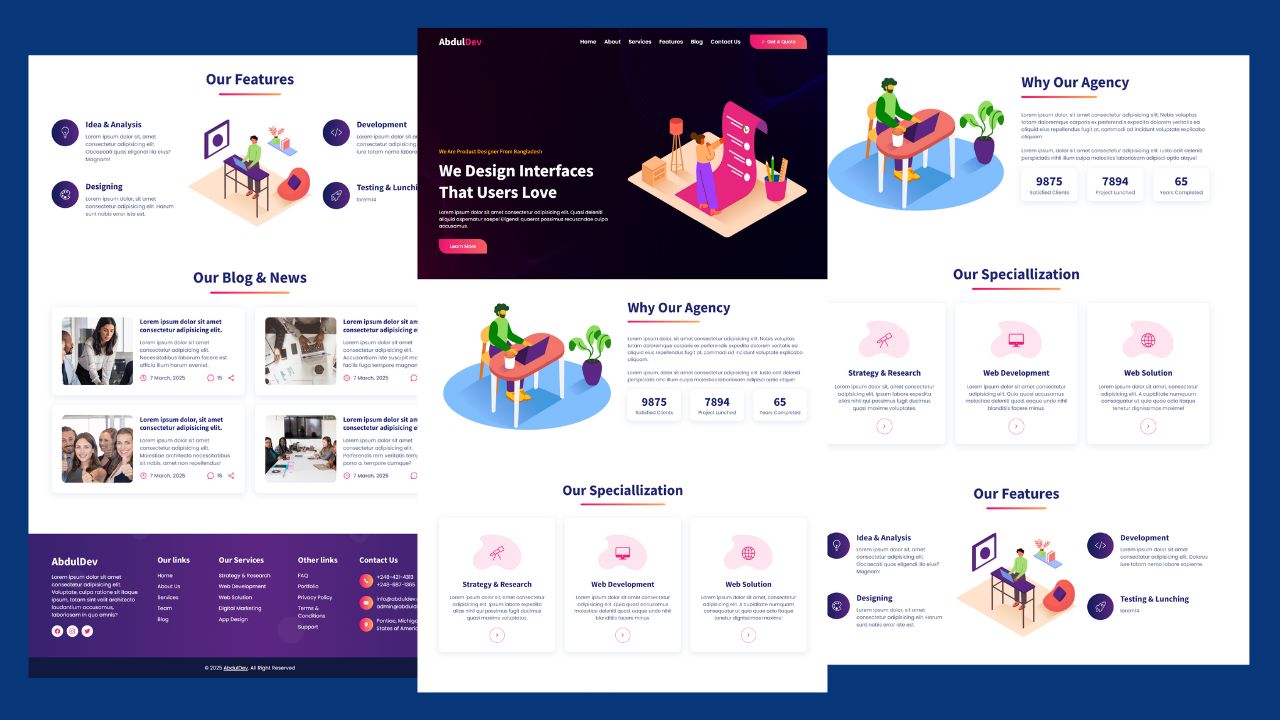
Creating a Fully Responsive Navigation Menu using HTML & CSS

I’m excited to share how to craft a fully responsive navigation menu bar using HTML and CSS. In a previous blog, I explored building a Responsive Registration Form for your website.
The navigation bar, or navbar, is integral to any website. It provides users easy access to different site sections and enhances overall navigation. With responsive design becoming increasingly crucial in today’s digital landscape, ensuring that your website’s elements adapt seamlessly to various screen sizes is essential.
In my design, as depicted in the preview image, we have a horizontal navbar with a logo positioned on the left and navigation links aligned to the right. This navigation bar is elegantly simple yet highly functional, and it’s achieved using only HTML and CSS.
One of the standout features of my navigation bar is its full responsiveness across all devices, including mobile phones. It appears as a horizontal bar on desktop screens, while on mobile devices, it transforms into a vertical layout. Users can easily toggle the menu by clicking the hamburger menu icon, providing a smooth navigation experience on smaller screens.
Responsive Navigation Menu Using HTML and CSS (Source Code)
To replicate this responsive navigation bar on your website, follow these simple steps:
- Begin by creating a folder for your project. You’re free to name this folder as you wish. Inside this folder, we’ll create the necessary files.
- Create a new file named index.html. The file name must be “index” with the extension “.html”.
- Next, create another file named style.css. Again, ensure the file name is “style” with the extension “.css”.
First, Copy the bellow HTML code and paste the codes into your index.html file.
Responsive Navigation Menu
Responsive Navigation Menu
Second, Copy the bellow CSS code and paste the codes into your style.css file.
*{
padding: 0;
margin: 0;
box-sizing: border-box;
}
body{
font-family: sans-serif;
}
nav{
background: #000048;
height: 90px;
width: 100%;
}
label.logo{
color: white;
font-size: 35px;
line-height: 86px;
padding: 0 40px;
font-weight: bold;
}
.logo span{
color: #df003b;
}
nav ul{
float: right;
margin-right: 40px;
}
nav ul li{
display: inline-block;
line-height: 80px;
margin: 5px;
}
nav ul li a{
text-decoration: none;
list-style: none;
color: white;
font-size: 17px;
padding: 7px 13px;
border-radius: 3px;
text-transform: uppercase;
}
a.active,a:hover{
background: #df003b;
transition: .5s;
}
.checkbtn{
font-size: 30px;
color: #df003b;
float: right;
line-height: 80px;
margin-right: 40px;
cursor: pointer;
display: none;
}
#check{
display: none;
}
@media screen and (max-width: 952px){
label.logo{
font-size: 26px;
padding-left: 40px;
}
nav ul li a{
font-size: 20px;
}
}
@media screen and (max-width: 858px) {
.checkbtn{
display: block;
font-size: 26px;
margin-right: 40px;
margin-top: 5px;
}
ul{
position: fixed;
width: 100%;
height: 100vh;
background: #09006d;
top: 80px;
left: -100%;
text-align: center;
transition: all .5s;
}
nav ul li{
display: block;
margin: 50px 0;
line-height: 10px;
}
nav ul li a{
font-size: 20px;
}
a:hover,a.active{
background: none;
color: #df003b;
}
#check:checked ~ ul{
left: 0;
}
}
.container{
width: 100%;
height: 86vh;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
background-image: url(bgimage4.png);
background-position: center;
background-repeat: no-repeat;
background-size: cover;
background-blend-mode: overlay;
background-color: #00000073;
}
.container h2{
color: #fff;
font-size: 45px;
font-weight: 600;
line-height: 55px;
text-align: center;
}
.container button{
margin-top: 30px;
padding: 15px 36px;
border: 2px solid #df003b;
border-radius: 45px;
font-family: 18px;
text-transform: uppercase;
font-weight: 600;
letter-spacing: 1px;
color: #fff;
background-color: #df003b;
cursor: pointer;
transition: all 0.6s ease;
}
.container button:hover{
background-color: transparent;
}
That’s it! Using HTML and CSS, you’ve successfully created a fully responsive navigation menu bar. Download the source code files from the provided link if you encounter any issues or errors while implementing the code. The download is free, and you’ll receive a .zip file containing all the necessary resources.
Enhance your website’s user experience and navigation with this sleek, adaptable, responsive menu bar. Happy coding!
FAQs
A responsive navigation menu bar is a user interface element on a website that adjusts its layout and design dynamically based on the size and resolution of the device screen. It ensures optimal viewing and navigation experience across various devices such as desktops, tablets, and mobile phones.
A responsive navigation menu bar is crucial for providing a consistent and user-friendly experience across different devices. It enhances usability, accessibility, and engagement by ensuring that visitors can easily navigate through the website regardless of the device they are using.
You can create a responsive navigation menu bar using HTML and CSS. By employing media queries, flexible layouts, and CSS techniques such as flexbox or grid, you can design a menu bar that adapts smoothly to various screen sizes.
Some best practices include keeping the menu simple and intuitive, using clear labels and icons, prioritizing important links, implementing touch-friendly design for mobile devices, and testing the responsiveness across different screen sizes and devices.
Yes, having a responsive navigation menu bar can positively impact SEO (Search Engine Optimization). Google considers mobile-friendliness as a ranking factor, so websites with responsive designs tend to rank higher in search results. Additionally, responsive design enhances user experience, which can lead to lower bounce rates and higher engagement, further boosting SEO.
Share on Social Media
Related Articles
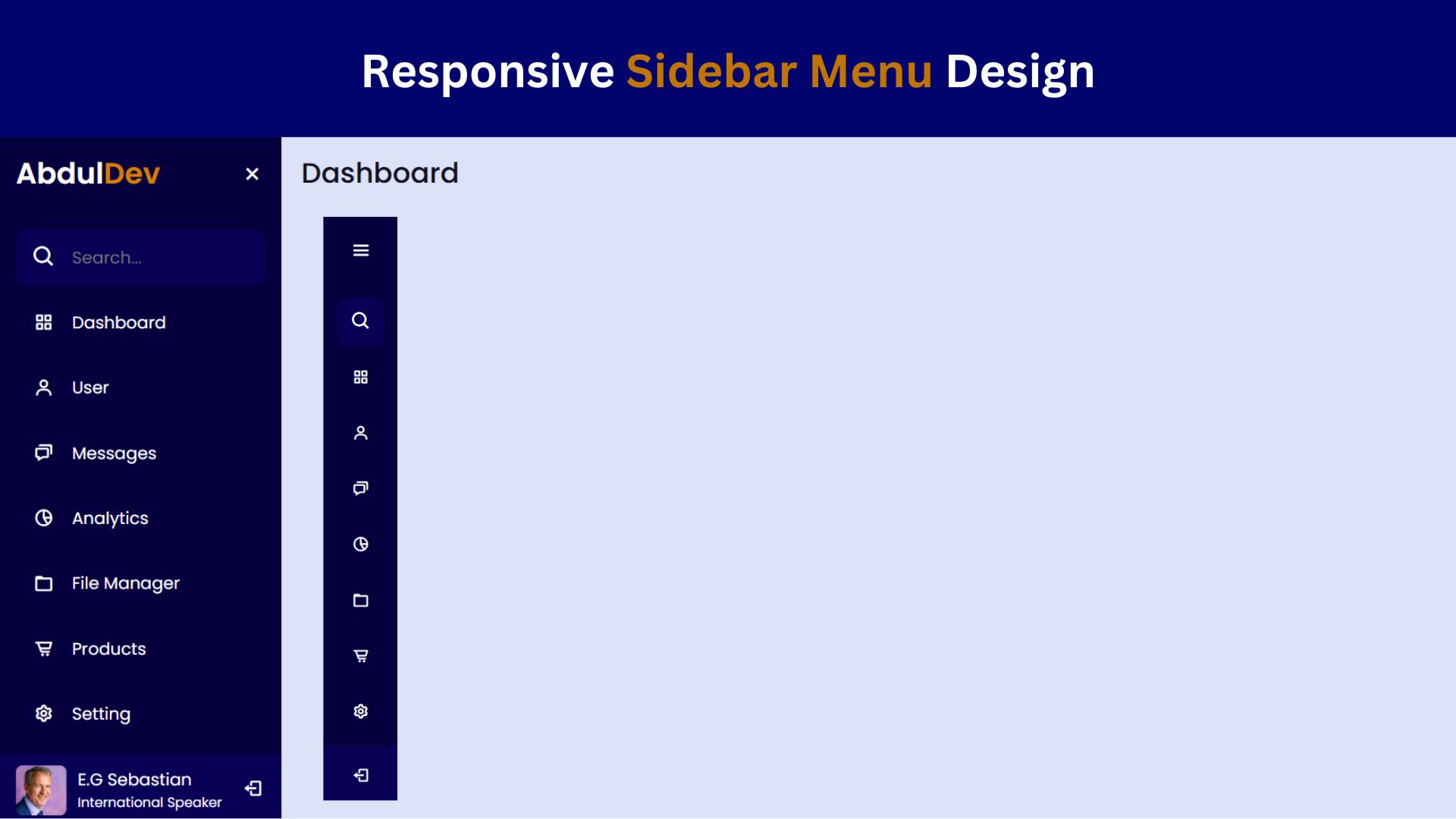
How to Create a Responsive Sidebar Menu Design
Learn how to create a responsive sidebar menu using HTML, CSS, and JavaScript. This step-by-step guide includes free source code
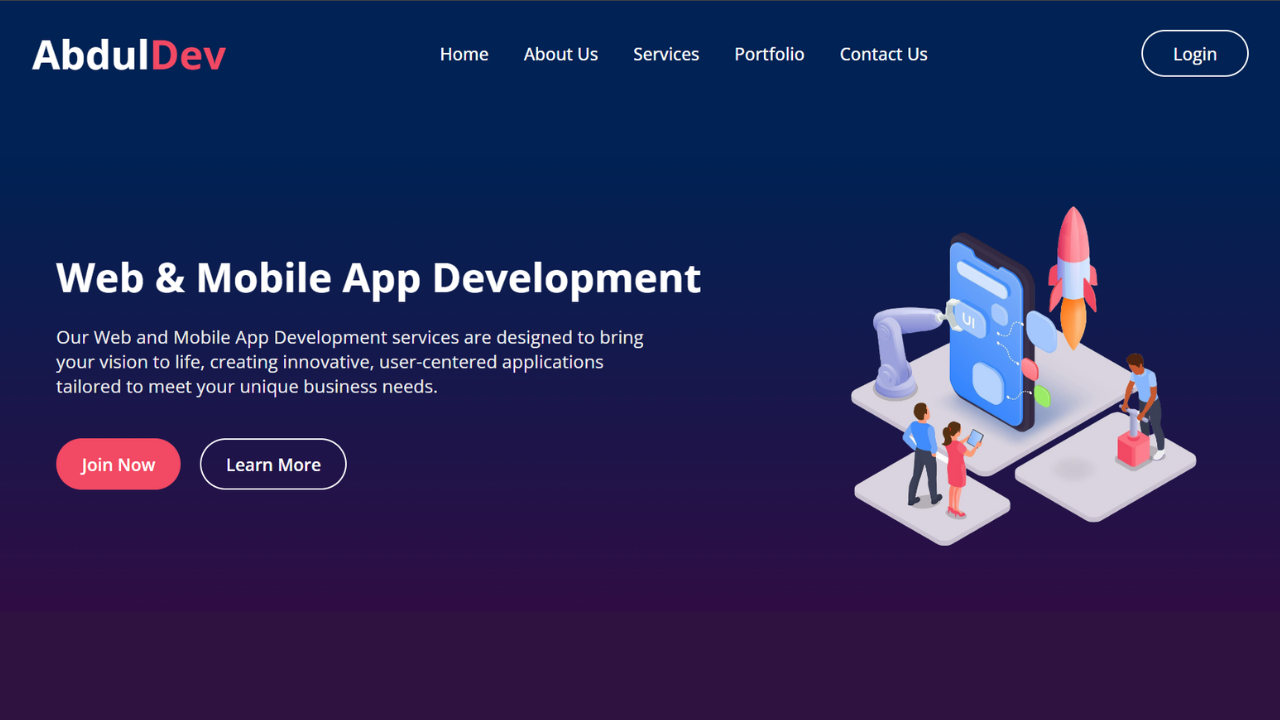
Responsive Website Homepage Design Using HTML and CSS
Learn how to create a responsive website homepage using HTML and CSS. This step-by-step guide covers mobile-friendly layouts, styling tips,

Responsive Dropdown Navigation Menu Using HTML, CSS, and JavaScript
Learn how to create a responsive dropdown navigation menu using HTML, CSS, and JavaScript! This step-by-step guide will help you
At AbdulDev, our mission is to support learners and developers by sharing helpful, easy-to-follow tutorials on modern web technologies like HTML, CSS, JavaScript, ReactJS, and PHP.
Useful Links
Copyright© 2025 | abduldev