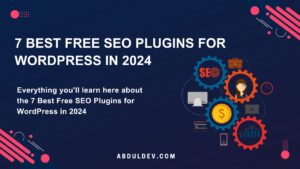
Responsive Registration Form Using HTML and CSS

If you’re eager to craft a responsive Registration Form using basic HTML and CSS, you’ve come to the right place!
This blog will guide you through the process of creating a Responsive Registration Form in HTML and CSS. Whether you’re just starting out or have a basic understanding of HTML and CSS, you’ll find this tutorial easy to follow.
A Registration Form is a crucial component of any website or application, where users must input their details such as name, email, address, and more.
Take a peek at the preview of the Registration Form you’ll learn to create in this blog. You’ll notice essential input fields every Registration Form should include, along with radio buttons for selection and a submit button.
If you’re keen to see the live demo of this Registration Form and explore all the HTML and CSS codes used to build it, check out the video tutorial below.
Registration Form Using HTML and CSS (Source Code)
Let’s walk through the steps to build a registration form using HTML and CSS:
- Begin by creating a folder for your project. You’re free to name this folder as you wish. Inside this folder, we’ll create the necessary files.
- Create a new file named index.html. The file name must be “index” with the extension “.html”.
- Next, create another file named style.css. Again, ensure the file name is “style” with the extension “.css”.
After you’ve set up your project folders, simply copy and paste the provided code snippets into their respective files. If you prefer not to do this manually, you can quickly download the HTML and CSS files for the registration form by clicking the download button below.
First, Copy the bellow HTML code and paste the codes into your index.html file.
Registration Form
Registration Form
Second, Copy the bellow CSS code and paste the codes into your style.css file.
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: sans-serif;
}
body{
min-height: 100vh;
display: flex;
align-items: center;
justify-content: center;
padding: 20px;
background: #0c0075;
}
.container{
position: relative;
max-width: 700px;
width: 100%;
background-color: #fff;
padding: 25px;
border-radius: 10px;
box-shadow: 0 0 15px #0000001a;
}
.container h2{
font-size: 24px;
font-weight: 600;
color: #333;
text-align: center;
}
.container .form{
margin-top: 30px;
}
.form .input-box{
width: 100%;
margin-top: 20px;
}
.input-box label{
color: #1e1e1e;
}
.form :where(.input-box input, .select-box) {
position: relative;
height: 50px;
width: 100%;
outline: none;
font-size: 16px;
color: #333;
margin-top: 8px;
border: 1px solid #ddd;
border-radius: 6px;
padding: 0 15px;
}
.input-box input:focus{
box-shadow: 0 1px 0 #0000001a;
}
.form .column{
display: flex;
column-gap: 15px;
}
.form .gender-box{
margin-top: 20px;
}
.gender-box h3{
color: #1e1e1e;
font-size: 18px;
font-weight: 500;
margin-bottom: 8px;
}
.form :where(.gender-option, .gender) {
display: flex;
align-items: center;
column-gap: 50px;
flex-wrap: wrap;
}
.form .gender{
column-gap: 5px;
}
.gender input{
accent-color: #0c0075;
}
.form :where(.gender input, .gender label) {
cursor: pointer;
}
.gender label{
color: #333;
}
.address :where(input, .select-box) {
margin-top: 15px;
}
.select-box select{
height: 100%;
width: 100%;
outline: none;
border: none;
color: #707070;
font-size: 16px;
}
.form button{
height: 50px;
width: 100%;
background-color: #0c0075;
color: #fff;
font-size: 16px;
font-weight: 500;
letter-spacing: 1px;
border: none;
cursor: pointer;
border-radius: 45px;
margin-top: 30px;
transition: all 0.6s ease;
}
.form button:hover{
background-color: #1101a2;
}
/* Responsive Code */
@media screen and (max-width: 500px) {
.form .column{
flex-wrap: wrap;
}
.form :where( .gender-option, .gender) {
row-gap: 15px;
}
}
If you encounter any challenges in building your Registration Form or if your code isn’t functioning as intended, you’re welcome to access the source code files for this Registration Form at no cost. Simply click the download button to get the files. Additionally, you can preview a live demo of this card slider by clicking on the view live button.
FAQs
A responsive registration form is a web form that dynamically adjusts its layout and design to fit different screen sizes and devices, ensuring optimal user experience across various devices like desktops, tablets, and smartphones.
Making a registration form responsive ensures that users can easily access and fill out the form regardless of the device they are using. It enhances user experience, reduces bounce rates, and increases conversion rates.
Basic knowledge of HTML and CSS is beneficial, but this tutorial is designed to be beginner-friendly, providing step-by-step instructions for creating the form. No prior experience is necessary, although familiarity with HTML and CSS will be helpful.
Yes, absolutely! The tutorial provides a foundation for building a registration form, and you can customize the design, colors, fonts, and layout to match your website’s style and branding.
Yes, you can easily add or remove fields according to your requirements. The tutorial covers the basics of creating the form structure, and you can extend it by adding more input fields as needed.
Share on Social Media
Related Articles
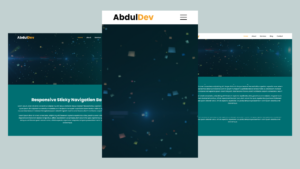
Responsive Sticky Navigation Bar Using HTML, CSS, and JavaScript
Learn how to create a responsive sticky navigation bar using HTML, CSS, and JavaScript. Follow our step-by-step guide with free
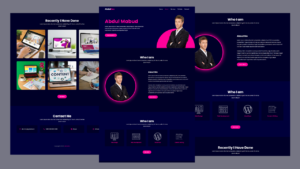
Creating a Responsive Portfolio Website with HTML, CSS, and JavaScript
Learn how to create a stunning and responsive portfolio website using HTML, CSS, and JavaScript. A step-by-step guide to showcase
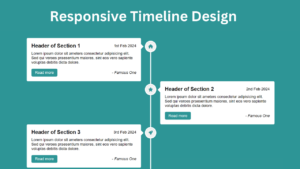
Responsive Vertical Timeline Design using HTML and CSS
Responsive Vertical Timeline Design using HTML and CSS Discover how to craft a Responsive Vertical Timeline Design utilizing HTML &