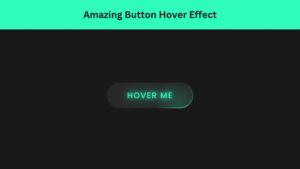
Build a Stunning Filterable Image Gallery Using Bootstrap
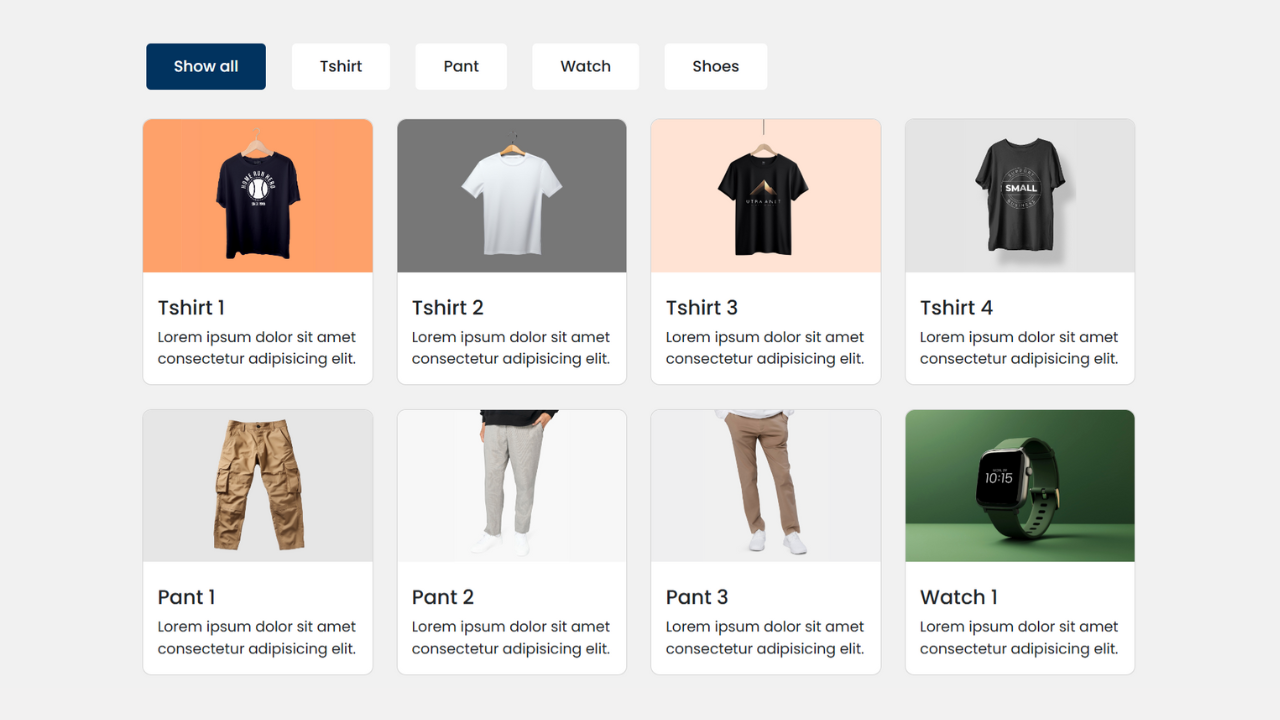
Creating a stunning filterable image gallery using Bootstrap is a fantastic way to showcase a collection of images in an organized and interactive manner. Whether you’re a photographer looking to display your portfolio or a business wanting to highlight product images, a filterable image gallery design can enhance user experience and improve engagement. This guide will walk you through building your image gallery with Bootstrap, making the process simple and enjoyable.
Understanding the Basics of Bootstrap
Bootstrap is a robust front-end framework designed to help you build responsive websites quickly and efficiently. It offers a wide array of pre-designed CSS and JavaScript components that enable you to create modern, user-friendly web interfaces without starting from scratch.Â
One of Bootstrap’s key features is its grid system, which allows you to design fluid layouts that adjust seamlessly across different screen sizes. This ensures your website remains accessible and visually appealing, whether viewed on a desktop, tablet, or smartphone.Â
Additionally, Bootstrap includes various utility classes that simplify everyday tasks like spacing, alignment, and typography, making the design process smoother and more intuitive.
Check Out Those Useful Articles
Setting Up Your Bootstrap Environment
Begin by downloading Bootstrap from its official website or including it via a CDN link in your HTML file. Ensure that both the Bootstrap CSS and JS files are linked correctly in your document to access the framework’s styles and scripts. This setup is vital for leveraging Bootstrap’s capabilities.
Steps to Build a Filterable Image Gallery
To Build a Filterable Image Gallery follow these steps:
- Create a Folder: Name this folder according to your preference. Inside this folder, you’ll need to set up the following files:
- Create an index.html File: This file should be named index with the .html extension.
- Create a style.css File: This file should be named style with the .css extension.
- Creat a main.js file:Â This file name should be main with .js extension.
These files will form the basis of your Filterable Image Gallery.
Designing the HTML Structure of the Gallery
Start by setting up the HTML structure of your image gallery using Bootstrap’s grid system. Create a container to hold your entire gallery. Inside this container, add a row element to arrange your images. Each image should be placed within a column, using Bootstrap’s col classes to define the width. For instance, you could use col-md-4 to display three images per row on medium-sized screens.
Assign data attributes to your images to enable filtering. Each image element should have a unique identifier or belong to a specific category. This way, when users select a filter option, the JavaScript code can quickly identify and display the corresponding images.
Additionally, incorporate Bootstrap’s responsive classes to ensure your gallery looks great on all devices. Using the img-fluid class for your images ensures they scale correctly within their parent containers. This structured approach keeps your HTML clean and makes managing and updating your gallery easier.
Now, open your code editor and set up a new HTML file, copy those HTML Codes and Paste those Codes on index.html file.
Bootstrap Filterable Image Gallery Design by AbdulDev
Tshirt 1
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Tshirt 2
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Tshirt 3
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Tshirt 4
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Pant 1
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Pant 2
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Pant 3
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Watch 1
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Watch 2
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Shoes 1
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Shoes 2
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Shoes 3
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Applying CSS Styles for Visual Appeal
While Bootstrap provides a solid foundation for styling, adding custom CSS can significantly elevate the visual appeal of your gallery. Customize hover effects and transitions to make interactions more engaging.Â
Use CSS to fine-tune spacing, alignment, and color schemes, ensuring a cohesive look that matches your brand or personal aesthetic. Consider adding subtle animations to make your gallery more dynamic. You can also customize the appearance of the filter buttons to make them stand out, using techniques like border-radius, box-shadow, and custom fonts.Â
By incorporating these CSS enhancements, you can create a filterable image gallery that is not only functional but also visually captivating.
After that, copy those CSS Codes and Paste those Codes on style.css file.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
* {
font-family: "Poppins", sans-serif;
}
body {
background: #f1f1f1 !important;
}
body .container {
max-width: 1140px;
padding: 70px 20px;
}
#filter-buttons button {
font-size: 17px;
font-weight: 500;
padding: 12px 30px;
border-radius: 5px;
background: #fff;
border-color: transparent;
margin-right: 20px !important;
margin-top: 20px !important;
transition: 0.5s !important;
}
#filter-buttons button:hover {
background: #01335f;
color: #fff;
}
#filter-buttons button.active {
color: #fff;
background: #01335f;
}
#filterable-cards .card {
width: 15.9rem;
border-radius: 10px;
overflow: hidden;
margin-right: 10px;
margin-bottom: 10px;
}
.card img{
width: 100%;
border-radius: 10px 10px 0 0;
transition: 0.5s;
}
.card img:hover{
transform: scale(1.1);
cursor: pointer;
}
.card .card-title{
font-size: 22px;
padding-top: 10px;
}
#filterable-cards .card.hide {
display: none;
}
@media (max-width: 600px) {
#filterable-cards {
justify-content: center;
}
#filterable-cards .card {
width: calc(100% / 2 - 10px);
margin: 0;
}
.card .card-title{
font-size: 18px;
padding-top: 10px;
}
.card .card-text{
font-size: 14px;
}
#filter-buttons button {
margin-right: 10px !important;
margin-top: 10px !important;
}
}
Implementing Filterable Categories with JavaScript
To implement filterable categories in your image gallery, you will utilize JavaScript to manage the visibility of images based on the selected category. Begin by assigning unique data attributes to each image, indicating its category. Next, create filter buttons that users can click to choose different categories. Attach event listeners to these buttons to trigger a function to filter the images when clicked.
In your JavaScript code, define a function that reads the data attribute of each image and compares it with the selected category. Use the `classList` property to add or remove classes that control the display of each image. For example, you can add a class like ‘hidden’ to images that do not match the selected category, hiding them from view. Conversely, remove the ‘hidden’ class from images that match the chosen category, making them visible.
To enhance user experience, consider adding animations to the filtering process. For instance, you can use CSS transitions to fade images in and out as they are filtered smoothly. This creates a more engaging and dynamic interaction for users.
By effectively leveraging JavaScript, you can create a robust and interactive filterable image gallery that allows users to easily navigate through different categories, enhancing your gallery’s overall usability and appeal.
Finally, copy those JavaScript Codes and Paste those Codes on main.js file.
// Select relevant HTML elements
const filterButtons = document.querySelectorAll("#filter-buttons button");
const filterableCards = document.querySelectorAll("#filterable-cards .card");
// Function to filter cards based on filter buttons
const filterCards = (e) => {
document.querySelector("#filter-buttons .active").classList.remove("active");
e.target.classList.add("active");
filterableCards.forEach(card => {
// show the card if it matches the clicked filter or show all cards if "all" filter is clicked
if(card.dataset.name === e.target.dataset.filter || e.target.dataset.filter === "all") {
return card.classList.replace("hide", "show");
}
card.classList.add("hide");
});
}
filterButtons.forEach(button => button.addEventListener("click", filterCards));
Optimizing for Performance and Accessibility
Optimizing your filterable image gallery for performance and accessibility is vital for providing an excellent user experience. Compress images to reduce file sizes and improve load times, which is especially important for users with slower internet connections. Implement lazy loading to delay the loading of images until they enter the viewport, further enhancing page speed.
Use semantic HTML to ensure your content is meaningful and more accessible for search engines and assistive technologies to understand. Adding ARIA (Accessible Rich Internet Applications) attributes to your elements can improve accessibility for users relying on screen readers. For instance, using `aria-label` or `aria-described by` can provide additional context for your images and buttons.
Ensure your gallery is keyboard navigable by allowing users to move through images and interact with filter buttons using the keyboard. Test your design with various screen readers to confirm all content is accessible and easily navigable.
Consider implementing responsive design practices to ensure your gallery looks great and functions well on all devices. Use media queries to adjust the layout for different screen sizes and ensure that text and interactive elements are appropriately sized and spaced for touch interactions on mobile devices.
By addressing these performance and accessibility aspects, you can create an inclusive, fast, and user-friendly filterable image gallery that provides a better overall experience for all users.
Testing and Debugging Your Image Gallery
Conduct thorough testing across multiple browsers and devices to ensure your filterable image gallery operates seamlessly. Start by verifying that your layout remains consistent and visually appealing regardless of screen size or browser type. Pay close attention to any discrepancies in spacing, alignment, or responsiveness.
Next, interact with all filter buttons to confirm they accurately display the corresponding images. This includes testing different categories and combinations to ensure images are present and correctly displayed.
Use browser developer tools to check for JavaScript errors. Open the console to identify and resolve any scripting issues affecting functionality. Also, test the performance of your gallery, primarily if you have implemented lazy loading. Ensure images load quickly as users scroll through the gallery.
In addition to functionality, evaluate your gallery’s accessibility. Navigate through the gallery using only the keyboard to confirm that all interactive elements are reachable and operable. Use screen reader software to ensure descriptive labels and alternative texts are appropriately conveyed to visually impaired users.
For a more comprehensive testing process, solicit feedback from a diverse group of users. Gather insights on usability, appearance, and any potential issues they encounter. This can help you identify and address problems you have missed.
By diligently testing and debugging your image gallery, you can provide all users with a polished, enjoyable experience.
Conclusion
By following this guide, you’ve learned how to set up your Bootstrap environment, design the HTML structure of your image gallery, and apply CSS styles to enhance its visual appeal. Using Bootstrap components, you’ve also implemented filterable categories with JavaScript and added interactive features like modals and carousels. Remember, optimizing for performance and accessibility ensures your gallery is user-friendly and inclusive. Thorough testing and debugging guarantee a seamless experience across all devices and browsers. Don’t hesitate to experiment with various styles and interactive elements to make your gallery unique. With these tools and techniques, you can confidently create a filterable image gallery that showcases your images effectively and captivates and engages your audience.
FAQs
What is a filterable image gallery in Bootstrap?
A filterable image gallery is a dynamic gallery where users can view images organized by categories or tags. Using Bootstrap’s responsive grid system and JavaScript filters, users can easily select categories to display only relevant photos.
What are the critical components needed to create a filterable gallery?
The key components include Bootstrap for the grid layout, buttons or tabs for the filter categories, JavaScript (or jQuery) for the filter functionality, and images organized by relevant tags or classes.
Can I use Bootstrap’s built-in grid system to create the gallery layout?
Bootstrap’s grid system is perfect for creating a responsive gallery layout. You can use col classes (like col-md-4) to define the number of columns based on screen size, allowing the gallery to adapt to different devices.
How do I add filter buttons for the gallery?
Create buttons or tabs with unique data attributes or IDs for each category. When clicking a button, JavaScript filters the images by showing only those associated with the selected category.
What JavaScript code is needed to make the gallery filterable?
You can use JavaScript or jQuery to add click events to each filter button. When clicked, a button hides images that don’t belong to the selected category and shows only the relevant ones.
How can I make the gallery responsive?
Bootstrap’s grid system is responsive by design. Ensure you’re using appropriate column classes (col-, col-md-, etc.) and test the layout on different screen sizes to adjust as needed for optimal responsiveness.
Can I add animation to the filter transitions?
Yes, CSS animations can be applied to image elements to add a fade or slide effect when switching filters. This improves the user experience by making transitions smoother.
How do I organize images into categories?
Assign each image an HTML class or data attribute that matches the category name. This will make it easy for JavaScript to identify and display images based on the selected filter.
Share on Social Media
Related Articles
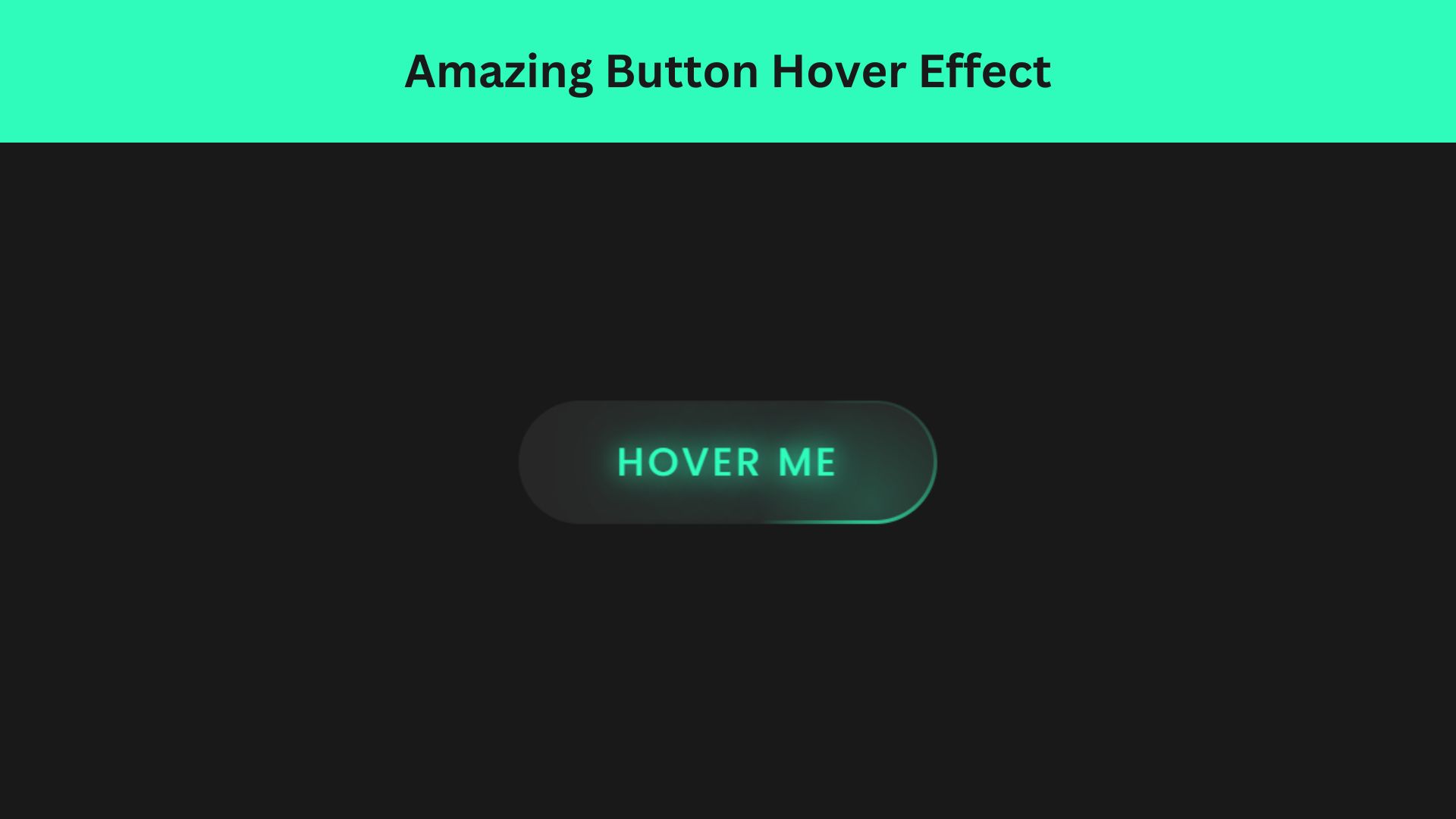
Amazing Button Hover Effect Using HTML, CSS, and JavaScript
Discover how to create amazing button hover effects using HTML, CSS, and JavaScript. Learn step-by-step with free source code to
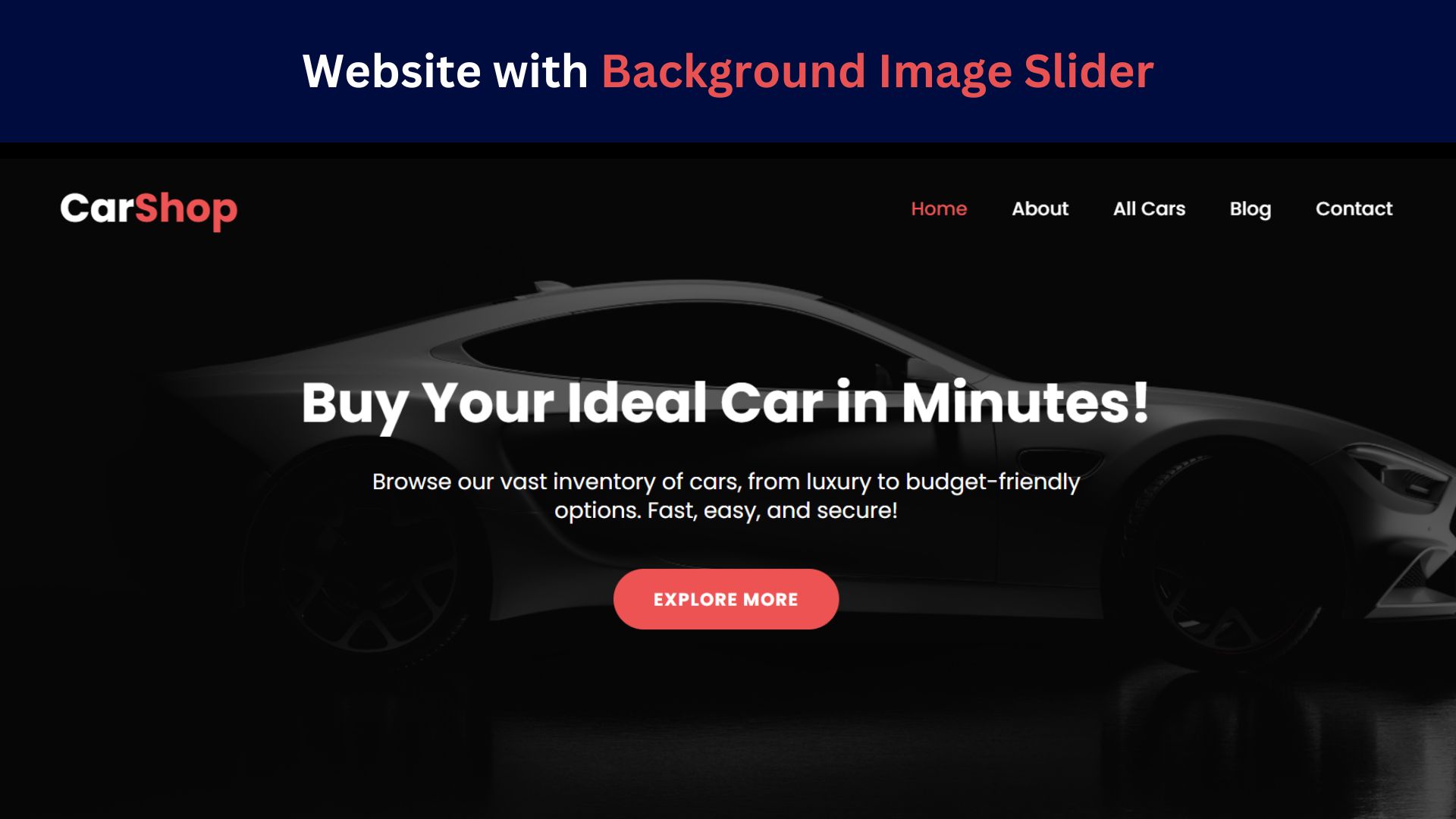
How to Design a Website with Background Image Slider Using HTML, CSS and JavaScript
Learn how to create a stunning, responsive background image slider for your website using HTML, CSS, and JavaScript. Follow our
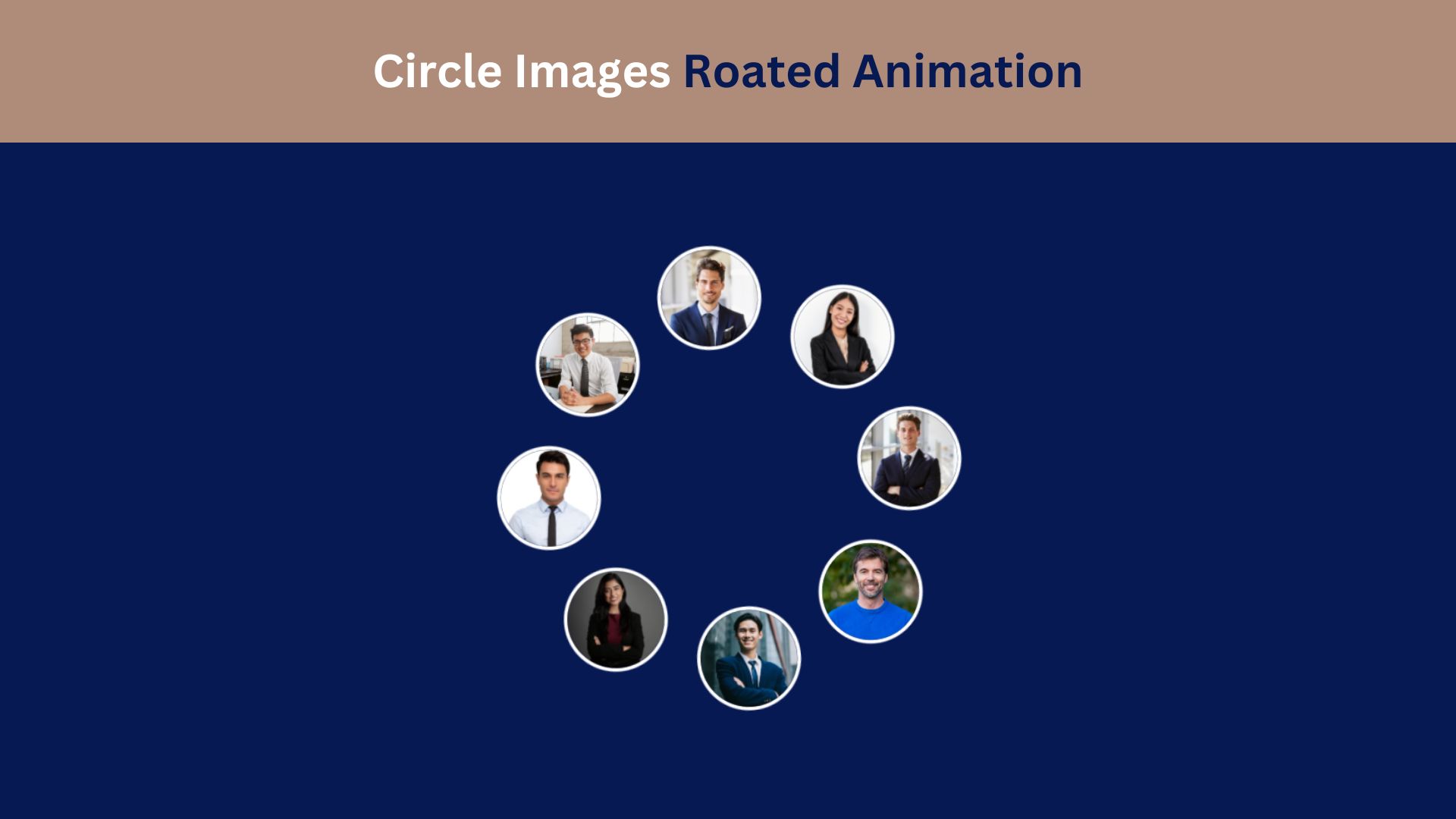
Circle Images Rotated Animation Using HTML and CSS
Learn how to create a captivating Circle Images Rotated Animation using HTML and CSS. This step-by-step guide helps you design