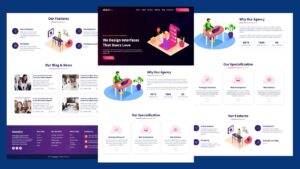
Responsive Dropdown Navigation Menu Using HTML, CSS, and JavaScript

A responsive dropdown navigation menu is an essential feature for websites, as it enhances user experience by organizing links in an intuitive way. In this tutorial, I’ll walk you through creating a simple and responsive dropdown navigation menu using HTML, CSS, and JavaScript. This guide will cover the basics, and by the end, you’ll be able to add this menu to your own projects.
What Is a Responsive Dropdown Navigation Menu?
A responsive dropdown navigation menu is a type of navigation that automatically adjusts its layout based on the user’s screen size. For larger screens, the navigation appears as a horizontal menu with dropdown options, while on smaller screens (like mobile devices), the menu typically collapses into a toggleable menu icon. This ensures that users can easily navigate regardless of their device.
Why Use a Responsive Dropdown Navigation Menu?
- Improved User Experience: A responsive menu provides an accessible and organized structure, making it easier for users to find content.
- Optimized for Mobile: With more users browsing on mobile, responsive menus are critical for a seamless experience.
- Enhanced Aesthetics: A dropdown menu gives a cleaner look, preventing clutter, especially on mobile devices.
Check Out Those Useful Articles
Steps to Create a Responsive Dropdown Navigation Menu
To Create a Responsive Dropdown Navigation Menu follow these steps:
- Create a Folder: Name this folder according to your preference. Inside this folder, you’ll need to set up the following files:
- Create an index.html File: This file should be named index with the .html extension.
- Create a style.css File: This file should be named style with the .css extension.
- Creat a main.js file: This file name should be main with .js extension.
These files will form the basis of your Responsive Dropdown Navigation Menu.
Setting Up HTML Structure
First, we’ll create the HTML structure of the menu. This code includes a basic navigation bar with menu items and a dropdown.
Responsive Drop Down Navigation Menu by AbdulDev
Styling with CSS for a Responsive Design
Now, let’s style our menu using CSS to ensure it’s responsive and visually appealing.
/* Googlefont Poppins CDN Link */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
min-height: 100vh;
}
nav{
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
height: 80px;
background: #02475e;
box-shadow: 0 1px 2px #00000033;
z-index: 99;
}
nav .navbar{
height: 100%;
max-width: 1250px;
width: 100%;
display: flex;
align-items: center;
justify-content: space-between;
margin: auto;
padding: 0 50px;
}
.navbar .logo a{
font-size: 36px;
color: #fff;
text-decoration: none;
font-weight: 600;
}
.navbar .logo a span{
color: #f8b391;
}
nav .navbar .nav-links{
line-height: 80px;
height: 100%;
}
nav .navbar .links{
display: flex;
}
nav .navbar .links li{
position: relative;
display: flex;
align-items: center;
justify-content: space-between;
list-style: none;
padding: 0 14px;
}
nav .navbar .links li a{
height: 100%;
text-decoration: none;
white-space: nowrap;
color: #fff;
font-size: 18px;
font-weight: 500;
}
nav .navbar .links li a:hover{
color: #f8b391;
}
.links li:hover .htmlcss-arrow,
.links li:hover .js-arrow{
transform: rotate(180deg);
}
nav .navbar .links li .arrow{
height: 100%;
width: 22px;
line-height: 80px;
text-align: center;
display: inline-block;
color: #fff;
transition: all 0.3s ease;
}
nav .navbar .links li .sub-menu{
position: absolute;
top: 70px;
left: 0;
line-height: 40px;
background: #02475e;
box-shadow: 0 1px 2px #00000033;
border-radius: 0 0 4px 4px;
display: none;
z-index: 2;
}
nav .navbar .links li:hover .htmlCss-sub-menu,
nav .navbar .links li:hover .js-sub-menu{
display: block;
}
.navbar .links li .sub-menu li{
padding: 0 22px;
border-bottom: 1px solid #ffffff1a;
}
.navbar .links li .sub-menu a{
color: #fff;
font-size: 17px;
font-weight: 500;
}
.navbar .links li .sub-menu .more-arrow{
line-height: 40px;
}
i.bx.bxs-chevron-right.arrow.more-arrow {
position: relative;
top: 2.5px;
}
.navbar .links li .sub-menu .more-sub-menu{
position: absolute;
top: 0;
left: 100%;
border-radius: 0 4px 4px 4px;
z-index: 1;
display: none;
}
.links li .sub-menu .more:hover .more-sub-menu{
display: block;
}
.navbar .search-box{
position: relative;
height: 40px;
width: 40px;
}
.navbar .search-box i{
position: absolute;
height: 100%;
width: 100%;
line-height: 40px;
text-align: center;
font-size: 26px;
color: #fff;
font-weight: 600;
cursor: pointer;
transition: all 0.3s ease;
}
.navbar .search-box .input-box{
position: absolute;
right: calc(100% - 40px);
top: 80px;
height: 60px;
width: 300px;
background: #02475e;
border-radius: 6px;
opacity: 0;
pointer-events: none;
transition: all 0.4s ease;
}
.navbar.showInput .search-box .input-box{
top: 65px;
opacity: 1;
pointer-events: auto;
background: #02475e;
}
.search-box .input-box::before{
content: '';
position: absolute;
height: 20px;
width: 20px;
background: #02475e;
right: 10px;
top: -6px;
transform: rotate(45deg);
}
.search-box .input-box input{
position: absolute;
top: 50%;
left: 50%;
border-radius: 4px;
transform: translate(-50%, -50%);
height: 35px;
width: 280px;
outline: none;
padding: 0 15px;
font-size: 16px;
border: none;
}
.navbar .nav-links .sidebar-logo{
display: none;
}
.navbar .bx-menu{
display: none;
cursor: pointer;
}
/* Responsive code for 920px */
@media screen and (max-width:920px) {
nav .navbar{
max-width: 100%;
padding: 0 25px;
}
nav .navbar .logo a{
font-size: 32px;
}
nav .navbar .links li{
padding: 0 10px;
white-space: nowrap;
}
nav .navbar .links li a{
font-size: 18px;
}
.navbar .links li .sub-menu a{
color: #fff;
font-size: 18px;
font-weight: 500;
}
}
/* Responsive code for 800px */
@media screen and (max-width:800px){
.navbar .bx-menu{
display: block;
}
nav .navbar .nav-links{
position: fixed;
top: 0;
left: -100%;
display: block;
max-width: 320px;
width: 100%;
background: #02475e;
line-height: 40px;
padding: 20px;
box-shadow: 0 5px 10px #00000033;
transition: all 0.5s ease;
z-index: 1000;
}
.navbar .nav-links .sidebar-logo{
display: flex;
align-items: center;
justify-content: space-between;
}
.sidebar-logo .logo-name{
font-size: 32px;
font-weight: 600;
color: #fff;
}
.sidebar-logo .logo-name span{
color: #f8b391;
}
.sidebar-logo i,
.navbar .bx-menu{
font-size: 40px;
color: #fff;
line-height: 80px;
}
i.bx.bx-x {
color: #ffffff;
line-height: 80px !important;
position: relative;
top: 7px;
left: 40px;
font-size: 40px;
cursor: pointer;
}
.search-box i.bx.bx-x {
color: #ffffff;
line-height: 80px !important;
position: relative;
top: -20px;
left: 0;
font-size: 34px;
cursor: pointer;
}
nav .navbar .links{
display: block;
margin-top: 20px;
}
nav .navbar .links li .arrow{
line-height: 40px;
}
nav .navbar .links li{
display: block;
}
nav .navbar .links li .sub-menu{
position: relative;
top: 0;
box-shadow: none;
display: none;
}
nav .navbar .links li .sub-menu li{
border-bottom: none;
}
.navbar .links li .sub-menu .more-sub-menu{
display: none;
position: relative;
left: 0;
}
.navbar .links li .sub-menu .more-sub-menu li{
display: flex;
align-items: center;
justify-content: space-between;
}
.links li:hover .htmlcss-arrow,
.links li:hover .js-arrow{
transform: rotate(0deg);
}
.navbar .links li .sub-menu .more-sub-menu{
display: none;
}
.navbar .links li .sub-menu .more span{
display: flex;
align-items: center;
}
.links li .sub-menu .more:hover .more-sub-menu{
display: none;
}
nav .navbar .links li:hover .htmlCss-sub-menu,
nav .navbar .links li:hover .js-sub-menu{
display: none;
}
.navbar .nav-links.show1 .links .htmlCss-sub-menu,
.navbar .nav-links.show3 .links .js-sub-menu,
.navbar .nav-links.show2 .links .more .more-sub-menu{
display: block;
}
.navbar .nav-links.show1 .links .htmlcss-arrow,
.navbar .nav-links.show3 .links .js-arrow{
transform: rotate(180deg);
}
.navbar .nav-links.show2 .links .more-arrow{
transform: rotate(90deg);
}
}
/* Responsive code for 420px */
@media (max-width:420px){
nav .navbar .nav-links{
max-width: 100%;
}
}
Adding Interactivity with JavaScript
Now, we’ll add JavaScript to toggle the menu’s visibility on smaller screens.
// search-box open close code
let navbar = document.querySelector(".navbar");
let searchBox = document.querySelector(".search-box .bx-search");
// let searchBoxCancel = document.querySelector(".search-box .bx-x");
searchBox.addEventListener("click", ()=>{
navbar.classList.toggle("showInput");
if(navbar.classList.contains("showInput")){
searchBox.classList.replace("bx-search" ,"bx-x");
}else {
searchBox.classList.replace("bx-x" ,"bx-search");
}
});
// sidebar open close code
let navLinks = document.querySelector(".nav-links");
let menuOpenBtn = document.querySelector(".navbar .bx-menu");
let menuCloseBtn = document.querySelector(".nav-links .bx-x");
menuOpenBtn.onclick = function() {
navLinks.style.left = "0";
}
menuCloseBtn.onclick = function() {
navLinks.style.left = "-100%";
}
// sidebar submenu open close code
let htmlcssArrow = document.querySelector(".htmlcss-arrow");
htmlcssArrow.onclick = function() {
navLinks.classList.toggle("show1");
}
let moreArrow = document.querySelector(".more-arrow");
moreArrow.onclick = function() {
navLinks.classList.toggle("show2");
}
let jsArrow = document.querySelector(".js-arrow");
jsArrow.onclick = function() {
navLinks.classList.toggle("show3");
}
Conclusion
Creating a responsive dropdown navigation menu is essential for modern web design. By using HTML, CSS, and JavaScript, we can create a user-friendly menu that adjusts to different screen sizes. Feel free to customize the colors, fonts, and layout to match your website’s branding. Adding a responsive dropdown menu improves both navigation and aesthetics, leading to a better user experience.
FAQs
What is a responsive dropdown navigation menu?
A responsive dropdown navigation menu is a flexible menu that adapts to different screen sizes. It appears as a horizontal menu on larger screens, while on mobile devices, it collapses into a toggleable icon (hamburger) for easy navigation.
Why is a responsive dropdown menu important for web design?
A responsive dropdown menu improves user experience by allowing users to easily navigate the site on any device. It keeps the interface clean, enhances accessibility, and is essential for mobile-friendly design.
How do I make a dropdown menu appear on hover?
In CSS, you can use the :hover
selector. For example, you can make a dropdown menu appear by setting .dropdown:hover .dropdown-menu { display: block; }
to show the submenu when the user hovers over the dropdown link.
What are the key CSS properties for styling a responsive menu?
Some key CSS properties include display
, position
, flex
, grid
, padding
, and background-color
. Media queries
are also essential for controlling how the menu behaves on different screen sizes.
Can I add a sliding effect to my dropdown menu?
Yes, by adding CSS transitions. For example, use transition: all 0.3s ease;
on the dropdown menu. This makes the menu appear and disappear smoothly rather than abruptly.
Is it possible to have multiple dropdown levels?
Absolutely! You can nest additional <ul>
elements within each dropdown item. Just ensure you style each level with unique classes to manage their appearance and behavior effectively.
Share on Social Media
Related Articles
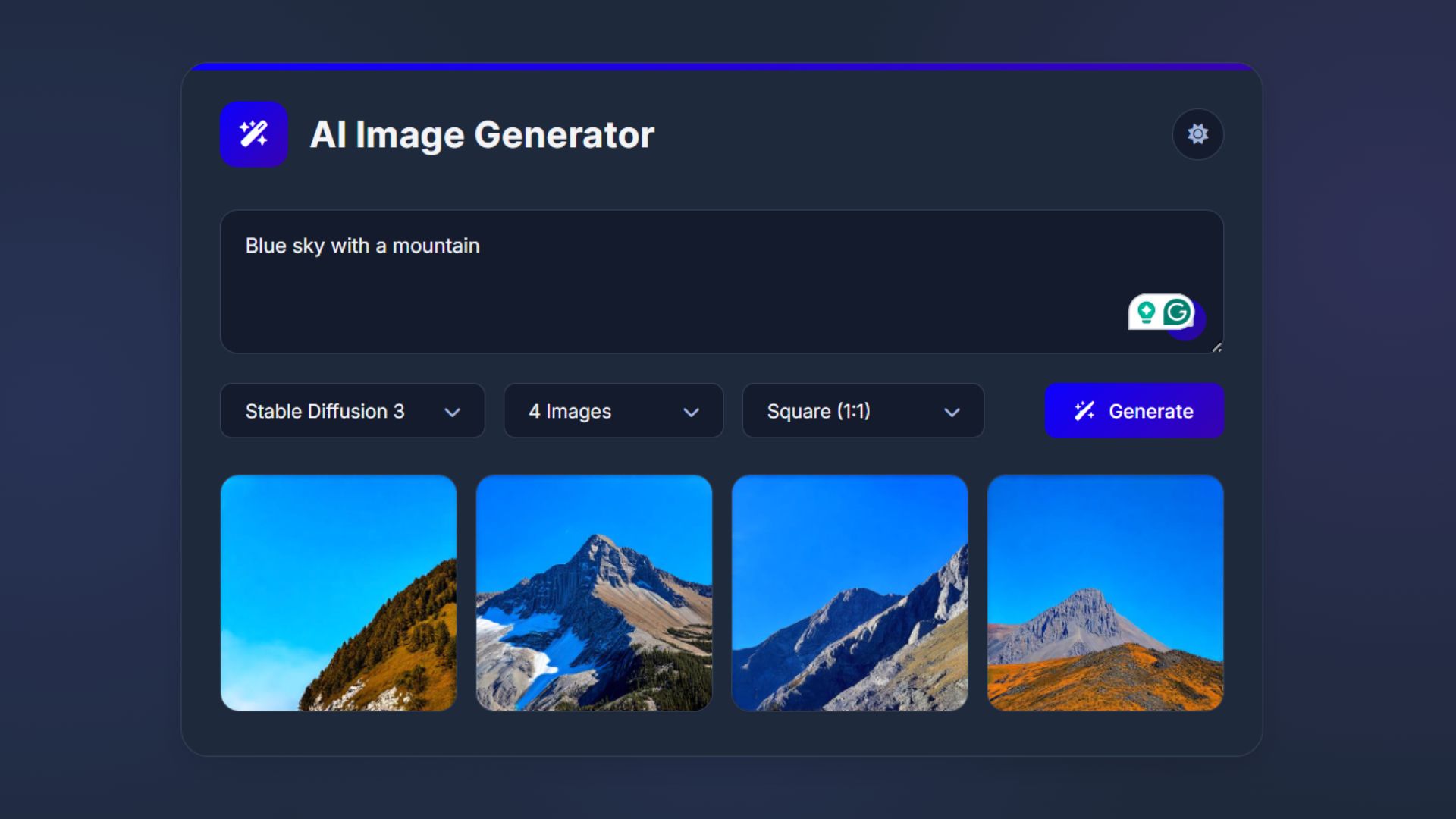
How to Build an AI Image Generator Using HTML, CSS, and JavaScript
Build a responsive AI Image Generator using HTML, CSS, and JavaScript. Learn how to integrate Hugging Face API to create

Build a Fully Responsive Sidebar with Dropdown Menu – HTML, CSS & JS
Learn how to build a fully responsive sidebar with dropdown menus using HTML, CSS, and JavaScript. Step-by-step guide with code
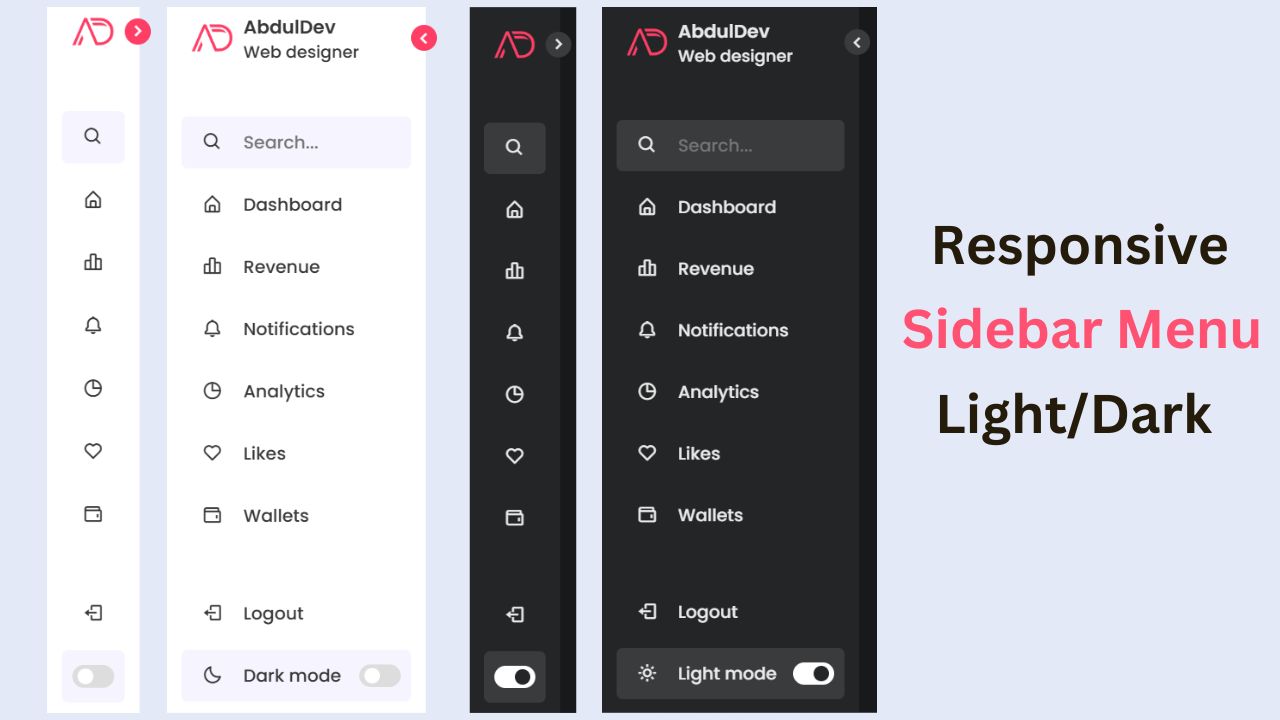
Responsive Sidebar Menu in HTML, CSS & JS | Light/Dark Mode
Learn how to create a modern responsive sidebar menu with a light and dark theme switcher using HTML, CSS, and
At AbdulDev, our mission is to support learners and developers by sharing helpful, easy-to-follow tutorials on modern web technologies like HTML, CSS, JavaScript, ReactJS, and PHP.
Useful Links
Copyright© 2025 | abduldev